Blogs & Social
BL0
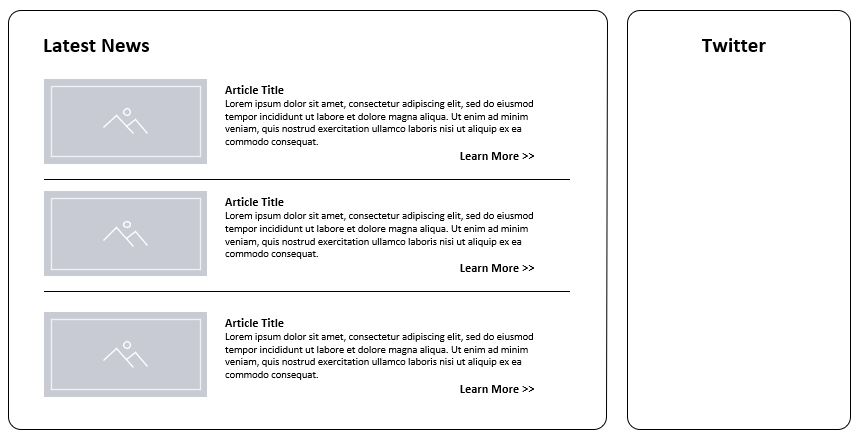
Code With ACF Integration
HTML
<!-- BL0 Start --------------------------------> <section id="wa_bl0"> <div class="container"> <?php // the query $args = array( 'post_type'=>'post', 'post_status'=>'publish', 'posts_per_page' => 3, 'paged' => get_query_var( 'paged' ) ); $wp_querys = new WP_Query( $args );?> <div class="row"> <div class="col-12 col-lg-9 mt-4"> <h2>Latest news</h2> <?php if ( $wp_querys->have_posts() ) : ?> <!-- the loop --> <?php while ( $wp_querys->have_posts() ) : $wp_querys->the_post(); ?> <div class="row"> <div class="col-12 col-lg-auto"> <?php $title = get_the_title(); $link_type = get_field('link_type'); if($link_type != 'none'){ if($link_type == 'internal'){ $link_url = get_field('internal_page_content'); }elseif($link_type == 'newpost'){ $link_url = get_the_permalink(); }elseif($link_type == 'external'){ $link_url = get_field('external_link'); $target = 'target="_blank"'; } $link = '<a href="'. $link_url.'" '.$target.'>'; $link_after = '</a>'; $read_more = '<a href="'. $link_url.'" '.$target.'><div class="read_more">Learn More</div></a>'; } $image = get_field('image'); if( !empty($image) ): ?> <?php echo $link; ?><img src="<?php echo $image['url']; ?>" alt="<?php echo $image['alt']; ?>" class="art_img" /><?php echo $link_after; ?> <?php else: ?> <?php echo $link; ?><img src="<?php echo get_site_url(); ?>/wp-content/plugins/webaika-news/img/default.jpg" alt="Web Aika Default Logo Icon" class="art_img" /><?php echo $link_after; ?> <?php endif; ?> </div> <div class="col-12 col-lg"> <?php echo '<h3>'.$link.$title.$link_after.'</h3>'; ?> <?php the_field('excerpt'); echo $read_more; unset($read_more); unset($link); unset($link_after); ?> </div> <?php if ($wp_querys->current_post +1 != $wp_querys->post_count) { echo '<div class="col-12"><hr class="post_hr"></div>'; } ?> </div> <?php endwhile; ?> <div class="wa_bl0_blog"> <a href="/blog">For more articles visit our blog</a> </div> <!-- end of the loop --> <?php wp_reset_postdata(); ?> <?php else : ?> <p><?php _e( 'Sorry, no posts matched your criteria.' ); ?></p> <?php endif; ?> </div> <div class="col-12 col-lg-3 mt-4"> <h2>Twitter Feed</h2> <div class="wa_bl0_twit"> <div class="wa_bl0_timg"> </div> <?php if ( is_active_sidebar( 'wa_tweeter' ) ) : ?> <div id="secondary-sidebar" class="new-widget-area"> <?php dynamic_sidebar( 'wa_tweeter' ); ?> </div> <?php endif; ?> </div> </div> </div> </div> </section> <!-- BL0 END -------------------------------->
CSS
/*-- BL0 Start ------------------------------------*/ @import url('https://fonts.googleapis.com/css2?family=Oswald:wght@200;300;400;500;600;700&display=swap'); #wa_bl0{ font-size: 15px; } #wa_bl0 h2{ font-size: 50px; font-family: 'Oswald', sans-serif; color: #cf9810; } #wa_bl0 h3 a:link, #wa_bl0 h3 a:visited, #wa_bl0 h3 a:active{ color: #000; } #wa_bl0 h3 a:hover{ color: #cf9810; } #wa_bl0 .read_more{ font-family: 'Oswald', sans-serif; color: #0979bd; font-size: 1.6em; } #wa_bl0 .read_more:hover{ color: #cf9810; } #wa_bl0 .wa_bl0_blog{ font-size: 1.3em } #wa_bl0 .wa_bl0_twit{ border: solid 2px #c7c7c7; border-radius: 5px; } .wa_twitter_widg .textwidget { padding: 10px; font-size: 13px; } .wa_bl0_categ{ height: 180px; position: relative; border: solid 1px #cf9810; border-radius: 5px; background-size: cover; } .wa_bl0_name{ position: absolute; bottom: 0; background-color: #000000b3; width: 100%; padding: 7px; text-align: center; color: #fff; font-weight: 600; font-size: 1.3em; border-top: solid 1px #fff; } .wa_bl0_categ:hover .wa_bl0_name{ transition: 1s; background-color: #ff5722f2; border-top: solid 1px #fff; } #wa_bl0 .wa_brcr{ background-color: #fff6de; border-bottom: solid 1px #03A9F4; font-size: 14px; padding-top: 5px; padding-bottom: 5px; } .art_img{ border: solid 2px #0e416c; border-radius: 5px; margin-top: 10px; margin-bottom: 20px; } .social_ul{ list-style: none; padding-left: 0; margin-left: 0; font-size: 30px; } .social_ul li{ display: inline-block; margin-right: 10px; } /* Pagination */ .navigation li a, .navigation li a:hover, .navigation li.active a, .navigation li.disabled { color: #fff; text-decoration:none; } .navigation li { display: inline; } .navigation li a, .navigation li a:hover, .navigation li.active a, .navigation li.disabled { background-color: #6FB7E9; border-radius: 3px; cursor: pointer; padding: 7px; font-size: 14px; } .navigation li a:hover, .navigation li.active a { background-color: #3C8DC5; } /*-- BL0 END ---------------------------------------*/
PHP
//---------------BL0 Start ---------------------------------------------------------------------------------// // Post pagination if ( ! function_exists( 'wpbeginner_numeric_posts_nav' ) ){ function wpbeginner_numeric_posts_nav() { if( is_singular() ) return; global $wp_query; /** Stop execution if there's only 1 page */ if( $wp_query->max_num_pages <= 1 ) return; $paged = get_query_var( 'paged' ) ? absint( get_query_var( 'paged' ) ) : 1; $max = intval( $wp_query->max_num_pages ); /** Add current page to the array */ if ( $paged >= 1 ) $links[] = $paged; /** Add the pages around the current page to the array */ if ( $paged >= 3 ) { $links[] = $paged - 1; $links[] = $paged - 2; } if ( ( $paged + 2 ) <= $max ) { $links[] = $paged + 2; $links[] = $paged + 1; } echo '<div class="navigation"><ul style="padding-left: 0; margin-left: 0;">' . "\n"; /** Previous Post Link */ if ( get_previous_posts_link() ) printf( '<li>%s</li>' . "\n", get_previous_posts_link() ); /** Link to first page, plus ellipses if necessary */ if ( ! in_array( 1, $links ) ) { $class = 1 == $paged ? ' class="active"' : ''; printf( '<li%s><a href="%s">%s</a></li>' . "\n", $class, esc_url( get_pagenum_link( 1 ) ), '1' ); if ( ! in_array( 2, $links ) ) echo '<li>…</li>'; } /** Link to current page, plus 2 pages in either direction if necessary */ sort( $links ); foreach ( (array) $links as $link ) { $class = $paged == $link ? ' class="active"' : ''; printf( '<li%s><a href="%s">%s</a></li>' . "\n", $class, esc_url( get_pagenum_link( $link ) ), $link ); } /** Link to last page, plus ellipses if necessary */ if ( ! in_array( $max, $links ) ) { if ( ! in_array( $max - 1, $links ) ) echo '<li>…</li>' . "\n"; $class = $paged == $max ? ' class="active"' : ''; printf( '<li%s><a href="%s">%s</a></li>' . "\n", $class, esc_url( get_pagenum_link( $max ) ), $max ); } /** Next Post Link */ if ( get_next_posts_link() ) printf( '<li>%s</li>' . "\n", get_next_posts_link() ); echo '</ul></div>' . "\n"; } } function register_wa_tweeter() { register_sidebar( array( 'id' => 'wa_tweeter', 'name' => esc_html__( 'Tweeter', 'webaika.com' ), 'description' => esc_html__( 'Tweeter feed', 'webaika.com' ), 'before_widget' => '<div id="%1$s" class="widget %2$s wa_twitter_widg">', 'after_widget' => '</div>', 'before_title' => '<div class="widget-title-holder"><h3 class="widget-title">', 'after_title' => '</h3></div>' ) ); } add_action( 'widgets_init', 'register_wa_tweeter' ); //---------------BL0 END ---------------------------------------------------------------------------------//
Images: 360px X 180px – Don’t need to be exact.
To Install:
- Download files bl0_files. Unzip files and upload to the theme.
- Open Functions file, and add functions that are located inside “PHP” window.
- Add CSS to the stylesheet.
- Open page template where you want BL0 to appear and add code inside HTML window.
- Download BLO Jason file, unzip and import within ACF plugin by going to “Custom Fields” > “Tools”
- Go to Plugins and install “Custom Twitter Feeds” by “Smash balloon” Activate.
- Go to “Appearance” > “Widgets” within the Tweeter widget add image and custom HTML. Image isn’t required. Within HTML add short code [custom-twitter-feeds]
- Go to “Twitter Feeds” and click “Log in to Twitter and get my Access token and secret”, Enter “feed type”, change number of tweets to display to around 3 and save.
- Add some posts, images and create categories.
- Go to “Pages” and create a new page. Title it “Blog” and select “Blog Page” from the page template selector.
A0090
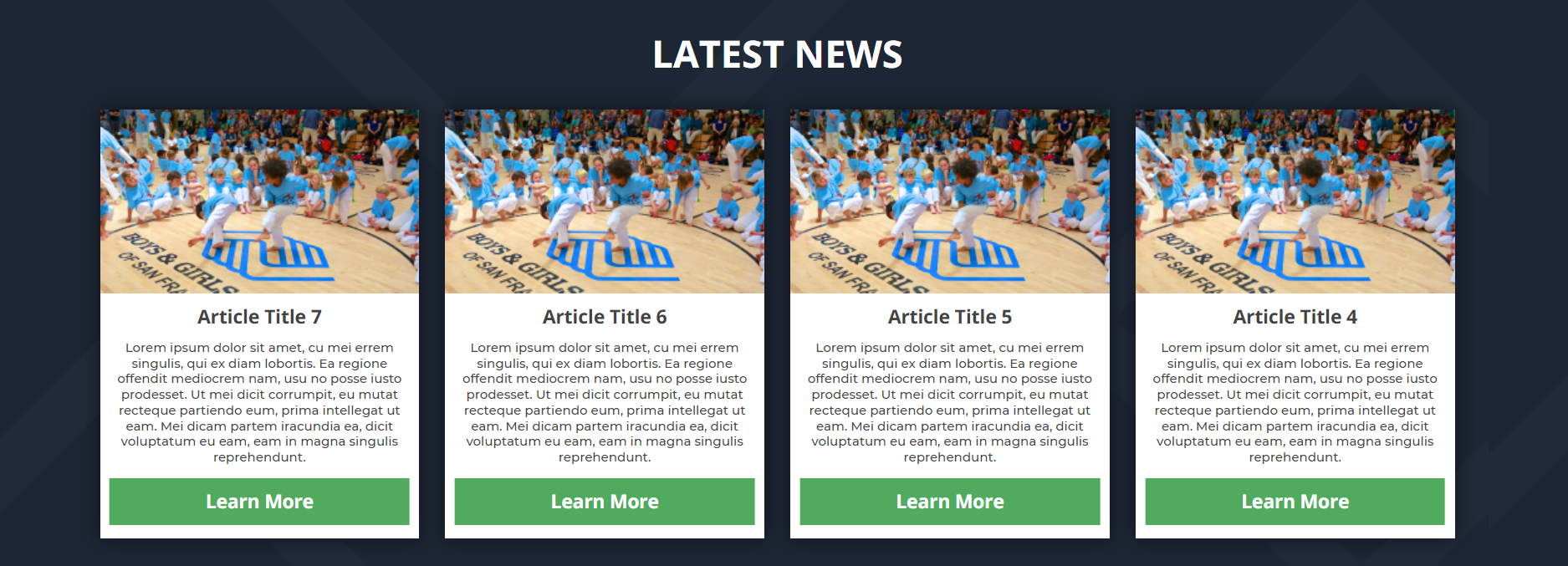
Code With ACF Integration
HTML
<!-- A0090 Start --------------------------------> <section id="a0090" style="background-image: url('<?php echo $tmp_dir."bg.png"; ?>')"> <?php // the query $args = array( 'post_type' => 'post', 'post_status' => 'publish', 'posts_per_page' => 4 ); $wp_querys = new WP_Query($args); ?> <div class="container"> <div class="row"> <div class="col-12"> <h2 class="a0090-header">Latest news</h2> <?php if ($wp_querys->have_posts()) : ?> <div class="a0090-grid"> <?php while ($wp_querys->have_posts()) : $wp_querys->the_post(); ?> <div class="a0090-item"> <?php $image = get_field('image'); $title = get_the_title(); $link_type = get_field('link_type'); if ($link_type != 'none') { if ($link_type == 'internal') { $link_url = get_field('internal_page_content'); } elseif ($link_type == 'newpost') { $link_url = get_the_permalink(); } elseif ($link_type == 'external') { $link_url = get_field('external_link'); $target = 'target="_blank"'; } $link = '<a href="' . $link_url . '" ' . $target . '>'; $link_after = '</a>'; } if (!empty($image)): ?> <?php echo $link; ?> <img src="<?php echo $image['url']; ?>" alt="<?php echo $image['alt']; ?>" class="a0090-art_img"/> <?php echo $link_after; ?> <?php endif; ?> <?php echo $link; ?> <h2><?php echo $title; ?></h2> <?php echo $link_after; ?> <div class="a0090-description"> <?php the_field('excerpt'); ?> </div> <a href="<?php echo $link_url; ?>" <?php echo $target; ?> class="a0090-read_more">Learn More</a> </div> <?php endwhile; ?> </div> <?php endif; ?> </div> </div> </div> </section> <!-- A0090 END -------------------------------->
CSS
/*-- a0090 ------------------------------------------*/ #a0090{ padding-bottom: 50px; } .a0090-grid{ display: grid; grid-template-columns: repeat(auto-fit, minmax(255px , 1fr)); grid-gap: 25px; } .a0090-header{ font-family: Open Sans; font-style: normal; font-weight: bold; font-size: 36px; line-height: 49px; text-align: center; text-transform: uppercase; color: #FFFFFF; margin-top: 50px; margin-bottom: 30px; } #a0090 img { width: 100%; } .a0090-item{ background: #FFFFFF; box-shadow: 0px 0px 20px rgba(0, 0, 0, 0.5); position: relative; padding-bottom: 70px; } .a0090-item h2{ font-family: Open Sans; font-style: normal; font-weight: bold; font-size: 18px; line-height: 25px; text-align: center; color: #434343; padding: 10px; margin: 0px; } .a0090-description{ font-family: Montserrat; font-style: normal; font-weight: 500; font-size: 12px; line-height: 15px; text-align: center; color: #434343; padding: 0px 5px; } .a0090-read_more{ background: #50AB5E; font-family: Open Sans; font-style: normal; font-weight: bold; font-size: 18px; line-height: 25px; text-align: center; color: #FFFFFF !important; width: 100%; display: block; padding: 10px; position: absolute; max-width: 94%; left: 50%; bottom: 13px; transform: translate(-50%, 0%); } @media (max-width: 768px) { .a0090-header { margin-top: 30px; } } /*-- a0090 END --------------------------------------*/
A0101
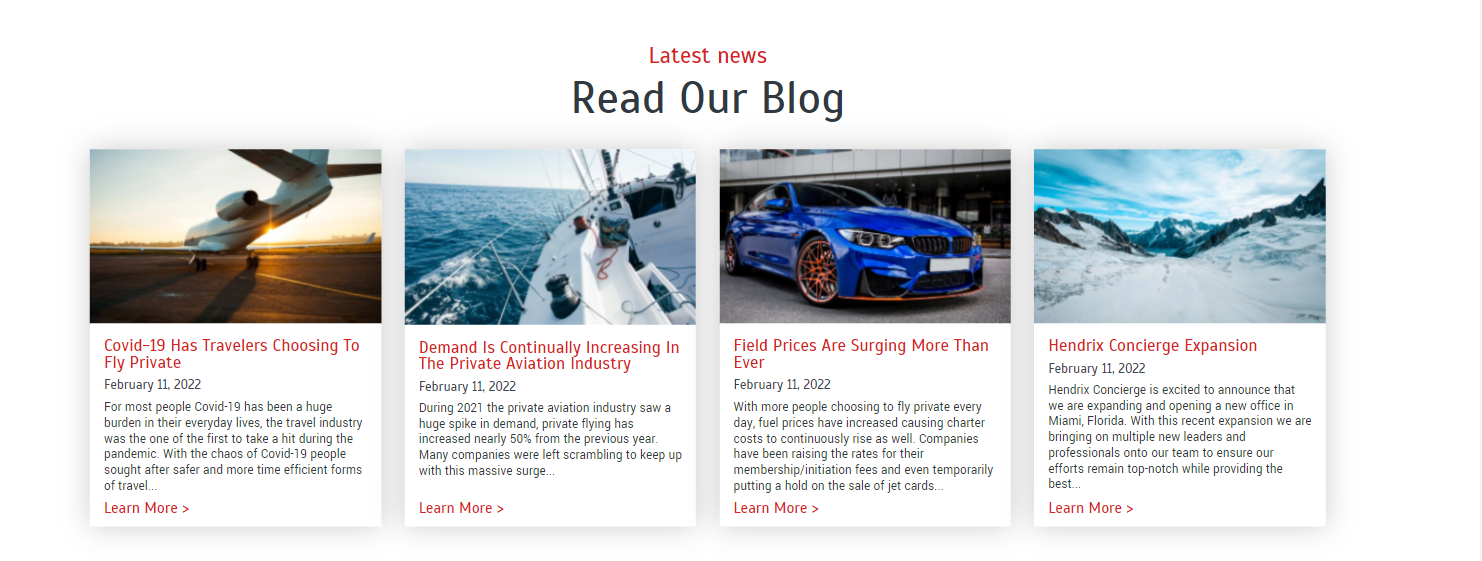
Code With ACF Integration
HTML
<!-- A0101 ----------------------------------------------------> <section id="a0101"> <div class="container"> <?php // the query $args = array( 'post_type' => 'post', 'post_status' => 'publish', 'posts_per_page' => 4, 'paged' => get_query_var('paged') ); $wp_querys = new WP_Query($args); ?> <div class="row"> <div class="col-12 col-lg-12 mt-4"> <h2>Latest news</h2> <h3>Read Our Blog</h3> <div class="a0101-grid"> <?php if ($wp_querys->have_posts()) : ?> <?php while ($wp_querys->have_posts()) : $wp_querys->the_post(); ?> <div class="a0101-item"> <?php $title = get_the_title(); $link_type = get_field('link_type'); if ($link_type != 'none') { if ($link_type == 'internal') { $link_url = get_field('internal_page_content'); } elseif ($link_type == 'newpost') { $link_url = get_the_permalink(); } elseif ($link_type == 'external') { $link_url = get_field('external_link'); $target = 'target="_blank"'; } $link = '<a class="a0101-lnk-head" href="' . $link_url . '" ' . $target . '>'; $link_after = '</a>'; $read_more = '<a href="' . $link_url . '" ' . $target . '><div class="read_more">Learn More ></div></a>'; } $image = get_field('image'); if (!empty($image)): ?> <?php echo $link; ?><img src="<?php echo $image['url']; ?>" alt="<?php echo $image['alt']; ?>" class="a0101-art_img" /><?php echo $link_after; ?> <?php else: ?> <?php echo $link; ?><img src="<?php echo get_site_url(); ?>/wp-content/plugins/webaika-news/img/default.jpg" alt="Web Aika Default Logo Icon" class="a0101-art_img" /><?php echo $link_after; ?> <?php endif; ?> <div class="col-12 col-lg a0101-pad"> <?php echo "<h6>".$link . $title . $link_after."</h6>" ; echo "<h5>".get_the_date()."</h5>"; ?> <?php the_field('excerpt'); echo $read_more; unset($read_more); unset($link); unset($link_after); ?> </div> </div> <?php endwhile; ?> <?php wp_reset_postdata(); ?> <?php endif; ?> </div> </div> </div> </div> </section> <!-- A0101 END ------------------------------------------------>
CSS
/*-- a0101 ------------------------------------------*/ #a0101{ margin: 40px 0px; } #a0101 h2{ font-family: Scada; font-style: normal; font-weight: normal; font-size: 24px; line-height: 100%; text-align: center; color: #D72323; margin-bottom: 10px; } #a0101 h3{ font-family: Scada; font-style: normal; font-weight: normal; font-size: 48px; line-height: 100%; align-items: center; text-align: center; color: #303841; margin-bottom: 30px; } .a0101-grid{ display: grid; grid-template-columns: 1fr 1fr 1fr 1fr; grid-gap: 25px; } .a0101-item{ background: #FFFFFF; box-shadow: 0px 0px 31px rgba(0, 0, 0, 0.2); position: relative; padding-bottom: 20px; } .a0101-art_img{ width: 100%; } .a0101-pad{ padding: 15px; font-family: Yantramanav; font-style: normal; font-weight: normal; font-size: 14px; line-height: 120%; color: #303841; } #a0101 h6 .a0101-lnk-head{ font-family: Scada; font-style: normal; font-weight: normal; font-size: 18px; line-height: 100%; color: #D72323; display: block; margin-bottom: 5px; } #a0101 h5{ font-family: Scada; font-style: normal; font-weight: normal; font-size: 14px; line-height: 100%; color: #3A4750; } .read_more{ font-family: Scada; font-style: normal; font-weight: normal; font-size: 16px; line-height: 20px; display: flex; align-items: center; color: #D72323; position: absolute; bottom: 10px; } @media (max-width: 1440px) { #a0101 h6 .a0101-lnk-head { font-size: 16px; line-height: 120%; } } @media (max-width: 1200px) { .a0101-grid { grid-template-columns: 1fr 1fr; grid-gap: 20px; } } @media (max-width: 768px) { #a0101{ margin: 20px 0px; } .a0101-grid { grid-template-columns: 1fr; } #a0101 h3 { font-size: 35px; margin-bottom: 20px; } } /*-- a0101 END --------------------------------------*/